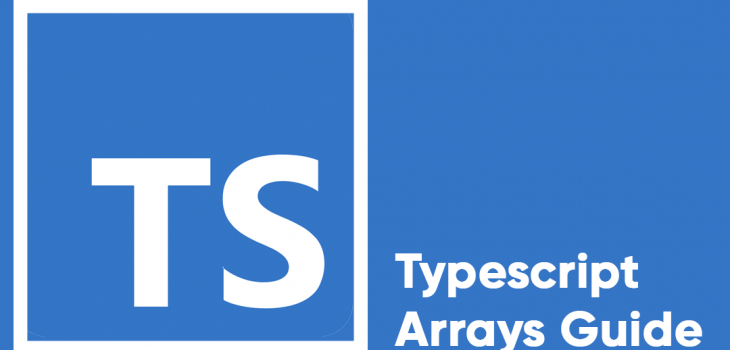
How to use Typescript with arrays
Array is used to store a sequence of elements as references. When declaring typescript array, the type of the elements should be defined in its syntax.
Read more “How to use Typescript with arrays”Array is used to store a sequence of elements as references. When declaring typescript array, the type of the elements should be defined in its syntax.
Read more “How to use Typescript with arrays” →React native always has been quite painful when it comes to performance compared to a native application. Today we will focus on increasing the performance and how to optimize react-native map view which has many pegs or markers.
Read more “How to optimize react-native map views” →Why debounce with react hooks? We all come across that one button users keep on pressing until the data is loaded. Without guarding your code with every sort of if conditions, you can guard this by debouncing. For multiple tap scenarios, you can either propagate the event at the first tap/click or the last one.
Read more “Avoid multiple clicks using debounce with react hooks” →Most of the developer’s idea is null checks makes the code less understandable and less reliable. Tony Hoare, who invented null references, calls them “a billion-dollar mistake”.
Read more “How to avoid NullPointerException in Java” →When you are building your react native Android app, you may encounter the error: heap limit Allocation failed.
FATAL ERROR: Ineffective mark-compacts near heap limit Allocation failed - JavaScript heap out of memory
Read more “Android/iOS React-native heap limit allocation failed error” → Recently, Java changed its release cycle more rapidly. JDK 13 released on September 2019 and JDK 14 is scheduled for a production release on 17 March 2020. The features targeted to JDK 14 as follows,
Java instanceof operator is used to test whether the object is an instance of the specified type. With Java 14 instanceof operator enhance to the pattern matching as bellow,
Read more “JDK 14 – The new features in Java 14” →This was an issue that was occurring after the migrating with new Androidx migration. The root cause was the android.support.v4.content.FileProvider
was not provided in the latest android support library.
To debug this error you will have to see the adb log
with the command
adb logcat
Read more “AndroidX ClassNotFound Exception: “android.support. v4.content .FileProvider”” → The numbers in Javascript has many subtle things to understand it fully. In this section, we are going to discuss the following points.
In this introduction to Javascript, we are going to discuss the following points.
Many newbies find AWS and domain providers such as GoDaddy confusing to connect. But it is not a difficult task and you can host GoDaddy domain with AWS EC2 instantly.
To connect AWS with the domain we will have to use a service called Route53 (53 refers thee TCP/UDP port 53 for DNS serving). And also there won’t be any direct way to do without this service. You can use any AWS public gateway provider such as EC2, ELB, S3 to serve your website or server to the public domain you have on GoDaddy. The AWS usually charges about .51 USD per month to maintain this zone entry as well.
Read more “How to host GoDaddy domain with AWS EC2” →Java Development Kit (JDK) 13, the latest version of standard Java, is now available and release with some new features of,
This is one of the preveiew language feature defined in java 13.
Java 13 extends the previous Java 12 Switch Expressions by adding a new yield keyword to return a value from switch expression.So breaks syntax is no longer compiled in Java 13, it uses yield instead.
This is another preview language feature defined in java 13. Java 13 extends the previous Java 12 Switch expressions by adding a new yield keyword to return a value from switch expression. So break syntax is no longer compiled in Java 13, it uses yield.
As traditional switch statement, there are many break statements make it unnecessarily verbose and hard to debug errors.
Read more “Java 13 Switch Expressions” →Nginx Load Balancer with docker has become a fine recipe for managing services in closed systems. Although services like Kubernetes provide container orchestration, sometimes architecturally it could be an overkill. In this example, I will be showing how to use docker services using Nginx load balancer.
Read more “Nginx Load Balancer with docker for nodejs services” →
Here we are discussing creating react app using npx create-react-app. npx comes with npm 5.2 and higher.
Prerequisite: You need to install Node >= 8.10 on your machine.
npx create-react-app my-app
create-react-app set up the main structure of the application.
Important: If you previously installed create-react-app using npm, uninstall before using npx. Because npx always refer the latest version of libraries.
npx comes bundled with npm version 5.2+.
If you want to run a package using npm, you must specify that package in your package.json file and install a package locally on a project:
npm install package-name
npx is a npm package runner.
This article will guide you on how to create a simple tab component using the react-tabs library.
Install react-tabs using
yarn add react-tabs
why yarn ?Let’s assume we are creating “helloWorld.jsx” like below, you can see there is a compilation error display as “’React’ must be in scope when using JSX react/react-in-jsx-scope.”
const element = <h1>Hello World!</h1> export default element;
This happens due to “React” import necessary in JSX file.The React library must also always be in scope from JSX code. To overcome this error “import React from “react”;” must be added into the file as follows.
Read more “The Common Errors that beginner React Developers Make” →
A preview feature or VM feature is a new feature of the Java SE Platform.
This article shows you how to use –enable-preview to enable the preview features in Java 13 with maven.
To use preview features in your programs, you must explicitly enable them in the compiler and the runtime system. Because this feature disabled by default.If not, you’ll receive an error message that states that your code is using a preview feature.
Read more “Enable the Preview Feature in Java 13 with Maven” →
Kubernetes has multiple services to facilitate the pod orchestration within the cluster. What we are discussing here is on how to use the AWS Ingress ALB with EKS without using the traditional Load Balancer Service
If you are new to firebase and FCM this article will be useful to setup FCM Push Notification with React Native application. We will be using react-native-firebase(doc: https://rnfirebase.io/docs/v5.x.x/getting-started) module to retrieve token for the push notifications.
First, create the Firebase account and download the google-services.json
file from the console.