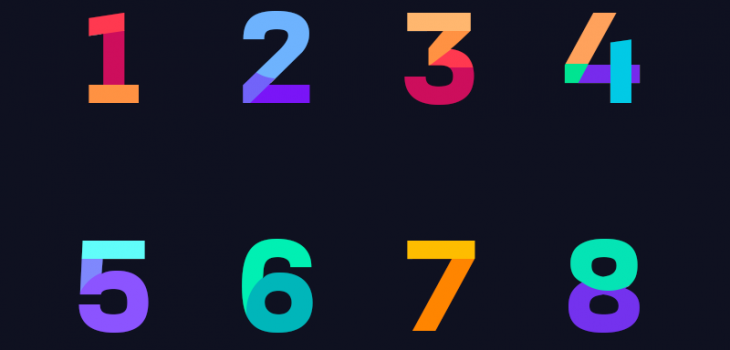
02. Javascript Guide – Numbers
Numbers in Javascript
The numbers in Javascript has many subtle things to understand it fully. In this section, we are going to discuss the following points.
- What are the numbers in Javascript and memory representation.
- Number wrapper.
- Arithmetics.
- Points to remember when coding.
1. Numbers
First thing, Javascript doesn’t have a separation between integers and floats as in many other languages.
It has one format which uses 64-bit floating point defined in IEEE 754 standard. We commonly term it as Double-precision format.
This can represent whole numbers from −9007199254740992 (−2^53) and 9007199254740992 (2^53).
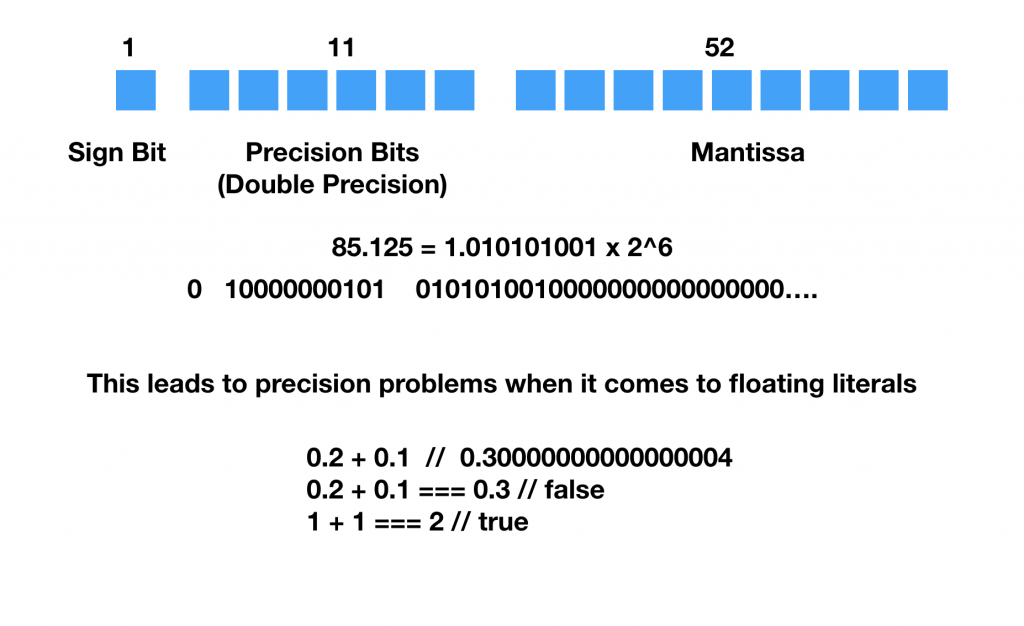
The way that double-precision floating literals are stored in the memory is in the above image.
It has one sign bit to represent positive and negative value. 11 precision bits and 52 mantissa bits. You can explore more about this format on https://en.wikipedia.org/wiki/IEEE_754
This format is not very precise. Floating points give an approximation of the decimal fraction value. Basically, when you add 0.2 with 0.1 it is not equal to 0.3.
This is a very important point to remember when you are working with sensitive data like money or scientific experimental values. Usually, we use decimal fractions as a whole number by scaling and arithmetic operations are performed on it.
Another option is to use libraries like Fraction to keep fraction value for the operations and construct the decimal fractions for display purposes only.
NaN
NaN represents not a number in Javascript. Any error operation with numbers will result in a NaN. NaN does not equal to infinity. Javascript has a predefined literal for it.
Some examples to produce NaN are;
0/0;
Infinity/Infinity;
parseInt('randomText');
NaN is not equal to itself since it doesn’t have a definite value.
NaN === NaN // false
An important thing to remember is, any erroneous arithmetic operation can lead to a result ending with NaN. So we will have to avoid such operations or executions as much as possible.
Infinity
Infinity is another number literal in javascript. This represents a positive value. Negative Infinity is represented with a negative sign. Some example to generate Infinity will be number divided by the zero.
And also just as NaN, typeof
operation gives Infinity as Number.
2. Number Wrapper
Wrappers are important concepts to grasp the Javascript language fully. The Number is the wrapper for floating literal in javascript. It is globally defined (you don’t have to require or import).
Floating literal are primitive values in javascript. It’s a value that has been allocated in a register as a value.
let num = Number(2) // 2
let primitiveNum = 2 // 2
To understand the wrappers, let’s take the following example.
let a = 10.20;
a.toFixed(); // 10
So how can it have a method like toFixed
? That’s when wrapper comes in. Calling a method on a literal a temporary wrapper it creates an object of Number
. Hence toFixed
method inherits from the number prototype.
This temporary object is immediately discarded once the execution is over.
You can find the supported methods and attributes from definitions like MDN. (https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Number)
3. Arithmetics with Numbers
Javascript supports common arithmetic operations in other languages.
*, /, +, -, %(Modulo, remainder after division), ++, --, +=,
-=
Apart from that, Math
global object can be used for any other need operations.
Remember there are no overflow or underflow errors in javascript.
Number.MIN_VALUE / 2 // 0
It doesn’t produce errors for some operations like minimum value divided by 2. It will simply result in the smallest value it can be produced which is zero.
4. Points to remember
Math.floor
This is an important operation. You can use it to extract the integer part of the number.
You can get Max, Min, Epsilon,
etc values from the wrapper.
Number.EPSILON
Number.MAX_SAFE_INTEGER
Number.MAX_VALUE
Number.MIN_SAFE_INTEGER
Number.MIN_VALUE
Number.NEGATIVE_INFINITY
Number.NaN
Number.POSITIVE_INFINITY
Number.prototype
Also, some functions like praseInt
, praseFloat, isNaN, isInfinity,
are widely used when programming with javascript.
parseInt("08") // 0
parseInt("08", 10) // 8
For praseInt
always use radix. Sometimes, leading zero can make it understand the value as an octal format. It will give you an unexpected result.
NaN, Infinity
are types of numbers you should always try to avoid them as much as possible.
And the most important one from them all. The precision of floating literals can give you unexpected values because of the way it is defined. Always use fractions or scale it to an integer value,
That’s it and I hope you got a good understanding of Numbers in Javascript.
In case you missed the introduction, check this one https://sandny.com/2019/12/11/01-javascript-guide-introduction-to-javascript/