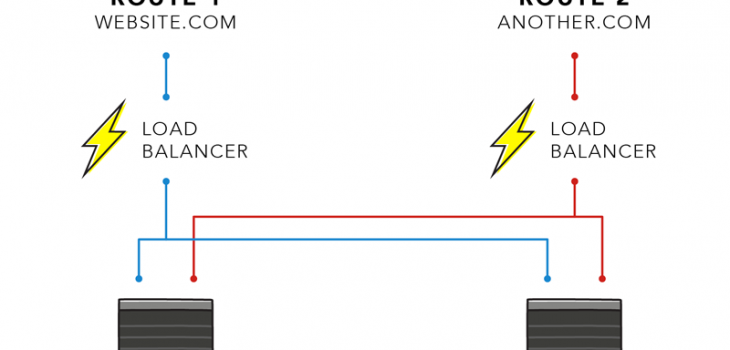
Nginx Load Balancer with docker for nodejs services
Nginx Load Balancer with docker has become a fine recipe for managing services in closed systems. Although services like Kubernetes provide container orchestration, sometimes architecturally it could be an overkill. In this example, I will be showing how to use docker services using Nginx load balancer.
Read more “Nginx Load Balancer with docker for nodejs services”