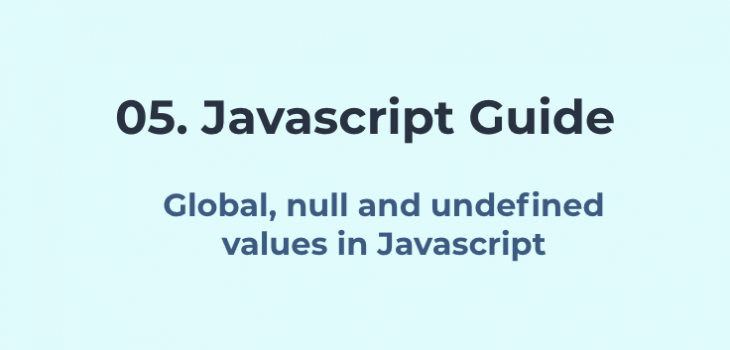
05 Global, null and undefined values in Javascript
Globals, undefined and null values are special concepts that we need to know when working with Javascript. Especially what both undefined and null means when you encounter it running the program. Globals are also an important concept that may give you an understanding of different attributes, the scope and variables that can be created and accessed when programming
1. null
value in Javascript
null
represents that a value is absent but it is done deliberately. It means that when programmatically you want to make clear a variable assignment best to use the null
value. By that when going through the next steps the programmer will understand that the null
value is explicitly set by a decision prior to that particular place. This is the main difference between undefined
and null
.
As an example
const user = {firstName: 'John', age: 18, relationships: null}; if (user.isRegistered === undefined) { // isRegistered is not defined in the object. // Which means that the object will not always consider having that property } if (user.relationships === null) { // this value is initialized to null. // which means it is set to null deliberately and that should be a property of the user }
The typeof null
returns an object which would represent that the null is a no object value. It can represent strings and numbers when there is no value as well. null
is also a reserved keyword in Javascript.
2. undefined
value in Javascript
The undefined value represents an uninitialized, unintended, or not considered value for different places.
For example:
When you define a variable without initializing to a value
let a; // not initialized console.log(typeof a === 'undefined'); // prints true console.log(a) // prints undefined
When you do not return any value from a function or pass a value to the parameter
const printNameWithoutReturn = (name) => { console.log(name) } const checkName = printNameWithoutReturn('Foo'); console.log(typeof checkName === 'undefined'); // prints true printNameWithoutReturn(); // prints undefined
undefined
is a read-only(ECMA5) global value rather than a keyword. The typeof undefined
returns 'undefined'
as a string which means that the language has defined this as a special type.
null and undefined are both falsy values which means
null == undefined // true null === undefined // false
There are no methods or wrappers for null
and undefined
. So dot .
operation or accessors []
would not work with those two as values or keywords.
3. isNil
function from Lodash
Sometimes people find it annoying to check both undefined and null in javascript without checking for falsy values. If Lodash or Underscore libraries are present, you can use a function like isNil, or create a global function that behaves like isNil to cover both scenarios. If it comes to this point we can assume that the code is not well structured but it can come in handy for large codebases with enhancements.(Don’t take a plus point to using isNil anywhere as conceptually it could go wrong in many cases. This is added in case you find it somewhere when coding)
For example:
var isNil = (value) => value === null || value === undefined; const user = {firstName: 'John', age: 18, relationships: null, balance: 0}; if (isNil(user.isRegistered)) { // isRegistered is not defined and it will proceed here } if (isNil(user.relationships)) { // isRegistered is defined as null and it will proceed here } if (user.balance) { // here the balance is 0 but its a valid value. // 0 is a falsy value for conditions and it will return a boolean when compared // hence its not accurate to test a number for the if condition } if (isNil(user.balance)) { // here it checks the balance is undefined or null // It will not proceed as the value is 0 }
4. Globals in Javascript
The language provides its own set of values, libraries, helpers, and different extensions for ease of use. This is what we refer to as the global object. Attributes of this object are globally defined for the javascript runtime it can be accessed everywhere.
For example:
- References
undefined
,NaN
,Infinity
- Functions
parseInt()
,encodeURI()
- Classes
Date
,String
,Array
- Object libraries:
Math
,JSON
For client-side (browsers mostly) the global object is defined as window
Example
window.parseFloat() this can be just accessed as parseFloat()
In the global scope where there isn’t any closures or not inside function or class, global can be accessed as this
keyword.
Example
this.parseFloat()
5. Declaring properties in the global scope
You can declare a variable in global scope and it cannot be deleted.
var globalProperty = 'I am a global property' delete globalProperty // returns false where it cannot be deleted. // You can set the value again
But you can always delete a variable that is initialized without var
in none “strict” mode
globalProperty = 'I am a global property' delete globalProperty // returns true where it can be deleted