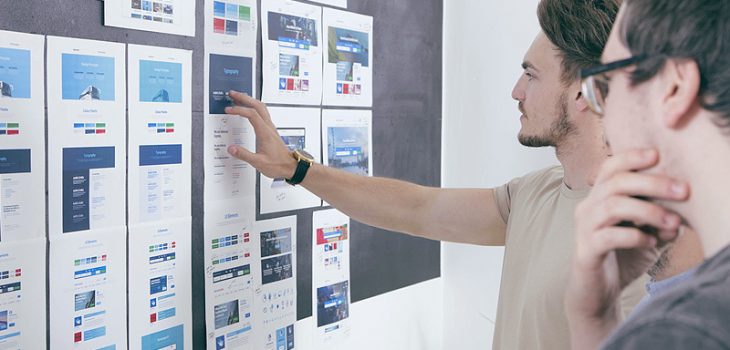
babel + express.js + node.js + nodemon to build…
This guide will take you through on how to use nodemon to build api which is simply to create a web app with node.js + express.js in a babel environment. Often we need to use babel to increase readability of our code. And nodemon was used to watch and re-build the project and serve it consistently.
Nodemon to build api
As a prerequisite, you will have to install the node.js and npm/yarn in your environment. I will be using yarn as it will make the . package maintenance easy. But I have included the npm command as well for your convenience.
First, initiate the project with
yarn init --------npm-------- npm init
Then install the project dependencies. I have run following in the terminal to install express(https://expressjs.com/), babel and nodemon(https://nodemon.io/).
yarn add express yarn add --dev babel-preset-env yarn add --dev nodemon --------npm-------- npm install express npm --save-dev babel-preset-env yarn --save-dev nodemon
The babel-preset-env
will install the presets es2015, es2016, es2017 along with some other(http://babeljs.io/docs/plugins/#presets). After that, you can add the script to start nodemon in the package.json
.
"scripts": { "start": "nodemon src/app.js --exec babel-node --presets babel-preset-env" },
Here babel-node
is provided with the babel-cli
. More of its usages are in the official doc: http://babeljs.io/docs/usage/cli/
Now all are set. We need to add the app.js file which I have specified in the script above. Create a filesrc/app.js
and add the hello world code mentioned below.
import express from 'express' const app = express(); app.get('/', function (req, res) { res.send('Hello World!') }); app.listen(3000, function () { console.log('Example app listening on port 3000!') });
Then run the app you have build. Run yarn start
on the terminal. You will see an output like below in the terminal.
$ yarn start yarn start v0.27.5 $ nodemon src/app.js --exec babel-node --presets babel-preset-env [nodemon] 1.12.1 [nodemon] to restart at any time, enter `rs` [nodemon] watching: *.* [nodemon] starting `babel-node src/app.js --presets babel-preset-env` Example app listening on port 3000!
The github link for the above project is: https://github.com/sandaruny/node-express-babel