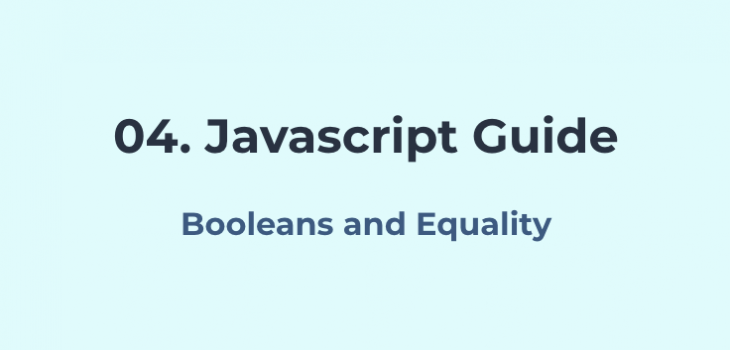
04. Javascript Guide Booleans and Equality
Javascript, just like any other language uses boolean to represent logical true or false. It has two reserved keywords true
and false
to represent a boolean value. Booleans are mostly comparative results as it will compare a value to another and check equality generally. Javascript compared to other languages have both strict and loose comparisons that we should mind when using. Lets start to explore more on Javascript Booleans and Equality
1. Javascript Booleans and Equality
A typical example of comparing a value in javascript is the following.
const a = 4, b = 5, c = 4, d = 'foo', e = '4';
console.log(a == b); // 4 == 5 -> false
console.log(a == c); // 4 == 4 -> true
console.log(a == d); // 4 == 'foo' -> false
console.log(a == e); // 4 == '4' -> true
console.log(a === e); // 4 === '4' false
If you are not familiar with equality operators ==
(loose) and ===
(strict) in JS, you might find it difficult to apprehend the above. We can discuss further it in the operators section in the future.
In above examples, a has value four and either of the sides matches with the value in equality it evaluates the result as true
while the contrary is false
. This is used to decisions and structures in programming languages and its the very fundamental of all programming languages. A typical if the condition is commonly used when programming as a control structure. Example
if (weather === 'SUNNY') {
openWaterValve();
} else {
startFans();
}
If the weather is sunny it will open the water valve and it will start the fans otherwise. This can be programmatically coded as above.
Also, all the javascript values can be converted to booleans and since javascript is loosely typed, its a convenient way to code. But it could also bring down very dramatic behaviors to codes and this should be really remembered when coding.
2. Truthy and Falsy values
Javascript has the following values which will convert to false when evaluated as a boolean.
undefined
null
0
-0
NaN
""
For example
const a = null, b = 0;
if (a || b) {
console.log('Someone is true');
} else {
console.log('Both are false');
}
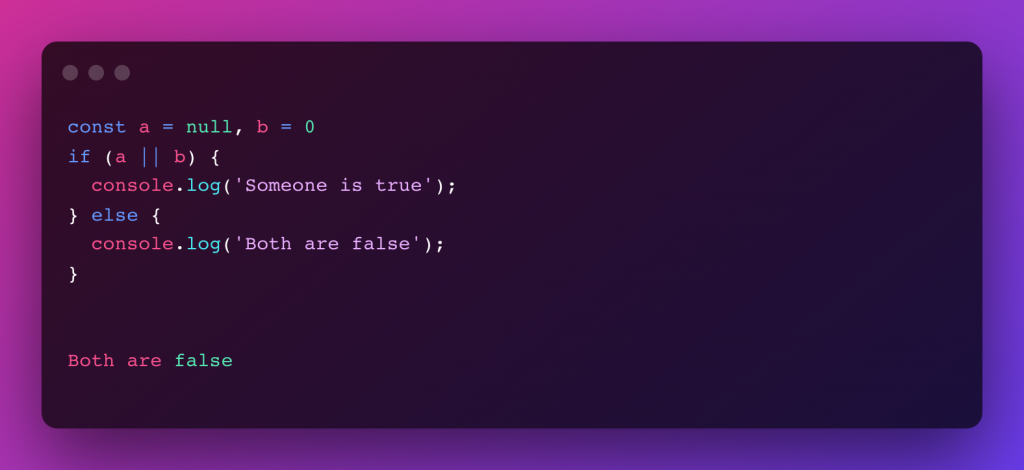
We call the above values that are not false but can be evaluated as false with a logical operation as falsy values. All the others including arrays, objects, functions etc. are known as truthy values. Its because they are not actually true or false but they act like that when converted.
Example:
const token = null;
if (token !== null) {
// fetch data
}
This can be coded as following as the null
value will be converted to false.
const token = null;
if (token) {
// fetch data
}
Note that this check is not strict. So if you assign any falsy value like null or undefined it will not proceed.
Other point is if you have an empty object {}
or empty array []
it will proceed as its not falsy.
const token = {}
if (token) {
// this will proceed
}
3. Boolean AND, OR operation
Boolean AND operation(&&) will proceed only if both sides of the operator are true or truthy. Most importantly it will execute a function only if the left hand side values are truthy before it comes to checking the function. As an example
const a = true, b = 1;
if (a && b) {
console.log('Both are true')
}
const user = isAuthenticated && getUser();
// isAuthenticated is evaluated first and if its only true, getUser() is called
Boolean OR (||) operation will proceed if any one of the conditions is true or truthy. It will go on checking every condition until it finds a true value otherwise. Important point is
- It will stop if as soon as it finds the true value when proceeding left to right
- I will continue until it finds a true value from left to right
One example you can code with this
const user = null;
// somewhere else
const loggedInUser = user || getUser(); // if user is null it will call get user
4. Unary !
operator
This performs a boolean NOT operation. Basically, if the value is truthy it will evaluate to false
and if the value is falsy it will evaluate to true
let user = null;
if (!user) {
user = fetchUser();
}
5. !! Not Not pseudo operator
This will convert the truthy or falsy value to true
and false
boolean values. This is not an operator this is a repetition of the !
operator. For example
const user = null;
const report = {
isUserAvailable: !!user, // resolves to boolean `false` value
}
6. ?? Nullish coalescing operator
This quite comes in handy when you want to use null assignments with ternary operators. It will return the right hand side value if the left hand side value is null
or undefined
only. For example
// null or undefined
const user = null;
const loggedUser = user ?? getUser(); // calls getUser()
// falsy values
const userCount = 0;
const loggedUserCount = userCount || getUserCount() // returns 0
This is different from the || operator. || the operator will return right side for all the falsy values. For the second example it will call getUserCount()
function although the value is already a number.
If you like to know more on javascript strings, check following link
Pingback: Globals undefined and null values in javascript when programming()