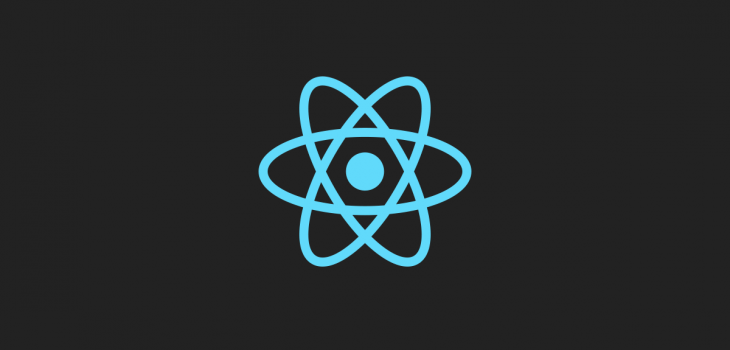
Using multiple refs for an array of React Elements
There are instances where you need to map elements and have multiple refs for an array of React Elements handled from higher-order components. For that and any similar circumstances, you can use the following approach to address this.
What is useRef
in React.js
According to the documentation from React.js
https://reactjs.org/docs/hooks-reference.html#useref
useRef
returns a mutable ref object whose.current
property is initialized to the passed argument (initialValue
). The returned object will persist for the full lifetime of the component.
Basically, the useRef
value has an attribute .current
that can hold a mutable value.
const Form = () => { const [inputRef] = useRef(null); const onPressStart = () => { inputRef.current?.focus(); }; return ( <> <button onClick={onPressStart}>Start Questionair</button> <input ref={inputRef} type="text" /> </> ); }
In the above example, the value of inputRef
.current is initialized to that of the <input>
element reference. By pressing the button it will focus on the input element.
Multiple refs for an array of React Elements
If we want to do this for several items in an array we can do the following. The useRef
the value will be initialized to an array of items and it will be updated using the useEffect
hook.
const inputArray = [1, 2, 3, 4]; // array of data const inputRefs = useState([]); const onPressStart = (index) => { inputRefs[index].current?.focus(); }; return ( <div> {inputArray.map((item, index) => ( <div key={index}> <h3>Question No: {item}</h3> <button onClick={() => onPressStart(index)}>Start Questionair</button> <input ref={element => inputRef.current[index] = element} type="text" /> </div> ))} </div> );
In the above code, the array of elements is stored in current and once the onPress is pressed it will focus on the input element. The index value is passed to the onPressStart
function to identify which element should be focused on when pressed on the button.