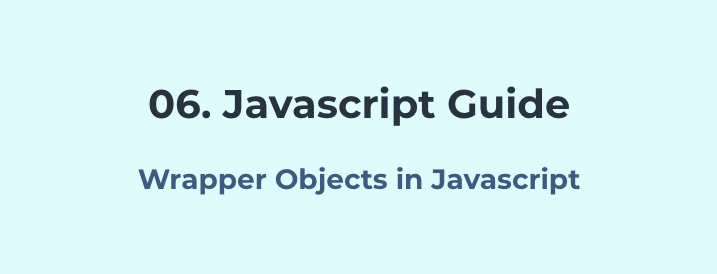
06. Wrapper objects in Javascript
Javascript provides many concepts under the hood. You may be using it without knowledge of its existence. Wrapper objects are one concept like that.
We know that javascript is object-oriented and we can create instances of objects and invoke it’s functions.
class Cat { sound = () => console.log('Meow...') } const cat = new Cat(); cat.sound(); // Meow...
In the above example, you can see that the Cat is a class that is a constructor function and you can call its method `sound()` by creating a variable.
Also if you could notice, you can invoke functions of string by just declaring it.
const aboutMe = "My name is: John"; const name = aboutMe.split(':')[1].trim(); const length = 'Hi there'.length();
You can see that in the above example the strings are not objects, but it inherits properties and methods of a `String` class. How does it happen?
This happens in Wrapper classes. It will create an object with `new String(str)` and then resolve the methods or properties when you access it. After that, it will be discarded. Same happens with `Object()` `Array`, `Function`, `Number()` `Boolean()` classes. The `null` and `undefined` values don’t have any wrapper classes
You can see this by the following example:
const str = 'Hi there'; str.myProperty = 'John' console.log(str.myProperty); // prints undefined
From this, we can derive it doesn’t refer to any object but rather it will create an object for certain property or method is accessed from the string literal. These objects are what we refer to as Wrapper objects.
Equality of Wrapper Objects
You can create objects out of Wrapper classes when necessary. They will be converted to primitive values when needed. The object and its value can be compared for equality but they would not be strictly equal to each other.
const str = 'Hello' const num = 12.2; strObj = new String('Hello') numObj = new Number(12.2) strObj === str // false strObj == str // true numObj === num // false numObj == num // true