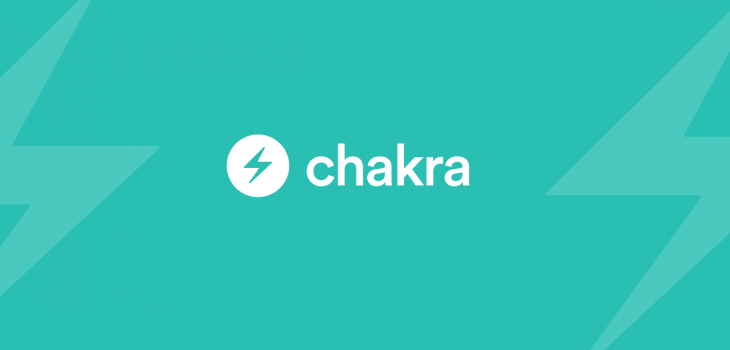
Chakra UI Animations
Chakra UI is becoming very popular as an HTML styling framework that allows you to style components in a more React way rather than css. One of the problems that people face is how to add animations to the React components using Chakra UI Animations.
Chakra UI has a parameter called animation
for the <Box>
and other logical containers such as <Flex>
, <Container>
If you look at the animation parameter it is the same as the CSS property animation
animation?: Token<CSS.Property.Animation>;
But animations that you have done in CSS has a keyframe attribute declared in their scope. For example
.slidein {
animation-duration: 3s;
animation-name: slidein;
animation-iteration-count: 3;
animation-direction: alternate;
}
@keyframes slidein {
from {
margin-left: 100%;
width: 300%;
}
to {
margin-left: 0%;
width: 100%;
}
}
Adding Chakra UI animations into a component
Like the above example, Chakra UI also has a method to define keyframe as a separate variable and inject into the scope of the component. For that, we need to import keyframe
from Chakra framework.
import { keyframes, usePrefersReducedMotion } from '@chakra-ui/react'
We also have imported one extra one. That is usePrefersReducedMotion
hook. It refers the x css property which provides animation preference of the user
The
https://developer.mozilla.org/en-US/docs/Web/CSS/@media/prefers-reduced-motionprefers-reduced-motion
CSS media feature is used to detect if the user has requested that the system minimize the amount of non-essential motion it uses.
So if the user has disabled animation to save resources, this variable will stop the animation.
Following is an example of using an animation
import { Image, keyframes, usePrefersReducedMotion } from '@chakra-ui/react'
import logo from './logo.svg'
const spin = keyframes`
from { transform: rotate(0deg); }
to { transform: rotate(360deg); }
`
const Example() => {
const prefersReducedMotion = usePrefersReducedMotion()
const animation = prefersReducedMotion
? undefined
: `${spin} infinite 20s linear`
return <Image animation={animation} src={logo} {...props} />
}
spin
variable that is globally declared and used as a formatted string.
Can you send animation-* css variables?
Currently, Chakra UI doesn’t support other variables individually. But you can pass all to the animation
parameter as following
/* duration | easing-function | delay | iteration-count | direction |
fill-mode | play-state | name */
animation={`3s ease-in 1s 2 reverse both paused ${slidein}`}
/* name | duration | easing-function | delay */
animation={`${slidein} 3s linear 1s`}
/* name | duration */
animation={`${slidein} 3s`}
Reference:
https://developer.mozilla.org/en-US/docs/Web/CSS/animation
https://chakra-ui.com/docs/hooks/use-prefers-reduced-motion