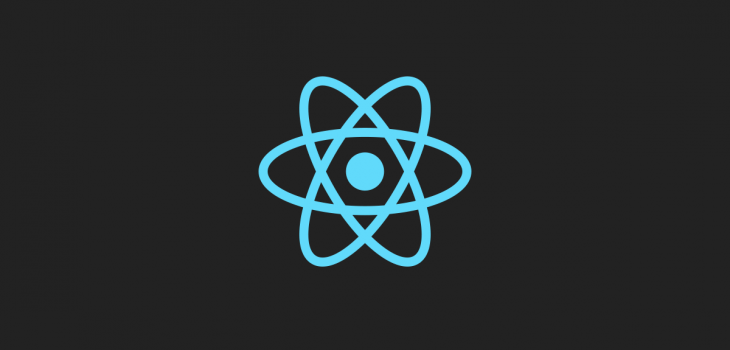
How to dismiss dropdowns when pressed on background with…
React components offer many advantages to break the code and implement web features. But when it comes to some features it could bit difficult to make it work. One such problem would be to listen to document click events and making the changes to components like dismissing dropdowns when pressed on the background.
1. How to listen to the click event globally
Using DOM global object document we can listen to the events of the root document. For that we can use addEventListener
function to add a callback function to DOM events.
const handleClick = (event) => { console.log('On click the document'); } document.addEventListener('click', handleClick); // to remove teh listener document.removeEventListener('click', handleClick);
2. Using useEffect to handle an event in component
We can utilize this DOM listener to handle click events and dismiss the Dropdown component.
For this we need to define a function to update the state. This state variable can be used to dismiss the dropdown.
const [showDropdown, setShowDropdown] = useState(false); const handleClick = useCallback(() => { showDropdown && setShowDropdown(false); }, [setShowDropdown]); useEffect(() => { document.addEventListener('click', handleClick); return () => { document.removeEventListener('click', handleClick); } }, [handleClick]); .... return ( <Dropdown onPress=(() => setShowDropdown(!showDropdown)) /> )
There are few important points in the above code.
The useCallback
is used to memoize and initialize the function once when the state is changed.
Then the useEffect
needs the event of callback variable to a dependency.
This will allow the showDropdown
state variable to be available in the scope of the callback function when the variable is updated. Unless the callback function always has the initial value of the showDropdown
state.
Then we need to remove the listener when the event is over. For that the removeEventListener
function will be returned as the callback from the useEffect
function.
You can find more information regarding the event listener from the MDN documents.
https://developer.mozilla.org/en-US/docs/Web/API/EventTarget/addEventListener