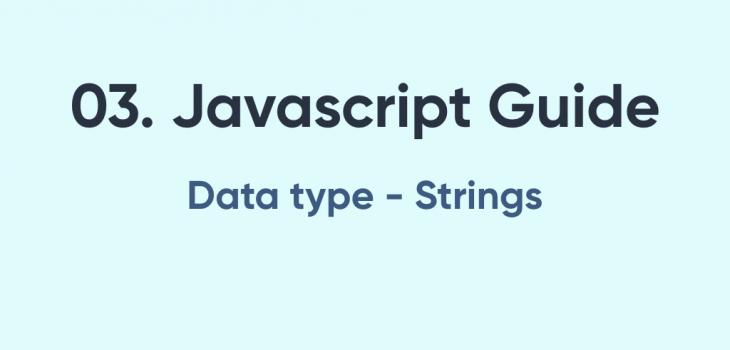
03. Javascript Guide – Strings
Every programming language has strings that represent a set of characters. Javascript strings have some properties which are unique to the language. This guide covers these properties and important facts when using strings.
1. What are strings?
In javascript, texts which can be assigned to a variable can be defined as strings. A string is
- Immutable sequence
- Sequence is ordered.
- Values of the sequence are 16-bit memory spaces, unicode characters.
The length of the string is how many these 16 bit values are present in the array.
Immutables means that when one string is defined that string cannot be mutated and the next one will be a next string. For example: if you define "hello"
and then "hello world"
, both of those memory locations will be separately defined in the memory space.
"hello ".concat("world"); // output => "hello world" // this doesnt change the orginal "hello " string and it will define a new string "hello world"
A string is a sequence 16-bit memory allocation and follows the standard of UTF-16. These strings are declared in javascript by enclosing between "",``, ''
Examples
"hello" === 'hello' === `hello` `hello ${user.firstName}!` "Hi, Foo" "two\nlines" 'string "inside string"' "" // empty string length is 0 "e\u0301" // é
After ECMA5 you can add multiline strings with the backslash(\)
"hello \ how are you" // output => "hello how are you" "hello how are you" // output => Uncaught SyntaxError: Invalid or unexpected token
Following is the representation of an ordered sequence of a string
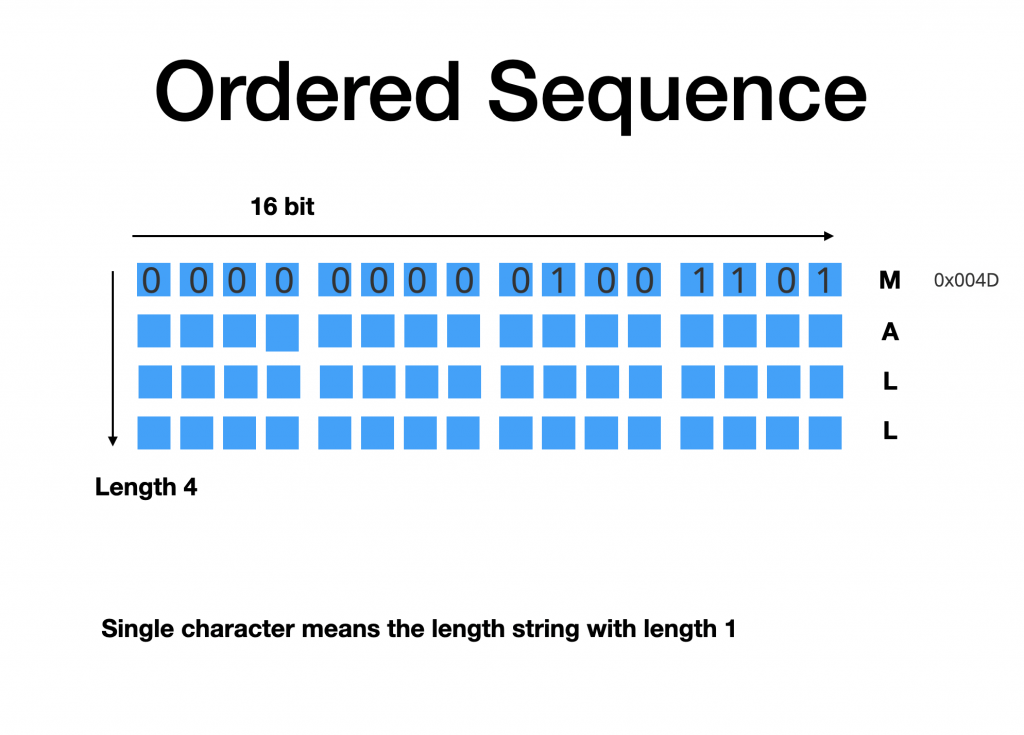
2. Surrogate Pairs
When we represent a character with 16 memory spaces there is a limit to which we can represent the characters(65,536). The characters which are in the basic multilingual plane can be represented in this 16 bits. This cannot represent all the characters in the language around the world and other special characters.
For that UTF-16 will extend the next set of the sequence and create a string of length 2.
Example
const a = "M" const b = "e\u0301" // é a.length // 1 b.length // 2
Hence this allows javascript to support all Unicode characters in when programming with strings.
3. String operations
You can perform many operations when programming with strings. Javascript support almost all of the string operations provided by the other popular languages.
Concatenate
"how " + "are you?"; "how ".concat("old are you?"); // string prototype function concat
Character string
We can use characters in the sequence by directly accessing it as an array variable.
const message = "hello "; message[0]; // h
Unlike programming languages like java, it won’t represent a single character or special character type to represent a sequence of characters or a similar approach in javascript.
Split strings
You can split an array of string by using the prototype function split.
"John, Foo".split(","); // ['John', ' Foo']
Escape literals
Another important operation is escaping the literals for special characters and literals. Following list show some of the common usages.
\n - new line
\b - back space
\v - vertical tab
\t - horizontal tab
\" - double quotes
\0 - End of character or nul character
\x - Latin 1 charcters 2 hexadecimal digits
\u - Unicode characters with 4 hexadecimal digits
4. String wrapper object
String is a wrapper object for string literals in Javascript. You can create a string also using String
constructor.
let hello = String("Hello world"); // => Hello world hello.toUpperCase();w
The toUpperCase()
the function is a prototype function that is provided by the String object.
When you call a method on a literal, temporary wrapper object of String is creating.
'hello'.toUpperCase();
You can find more information regarding String object in following reference.
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/String
5. Regular Expressions
Regular expressions in javascript are handled with RegExp
object. This is not a fundamental type object in javascript. But we can define a regexp using literal syntax and create a wrapper object directly when initiating. A RegExp can be paired in between two slashes as \expression\
Examples
let exp1 = /\w+/ let exp2 = new RegExp('\\w+') let re = /(\w+)\s(\w+)/ let str = 'John Smith' let newstr = str.replace(re, '$2, $1') // printes "Smith John"
Regular expressions are very important when doing string-related operations. As a programmer, you will be able to simplify many string operations if you know how to handle regular expressions properly in any language.
This covers most of the important aspects of Strings in javascript.
You can also refer to the previous tutorial on numbers to learn more about javascript language
Pingback: 04. Javascript Guide Booleans and Equality - Sandny Blog()
Pingback: Globals undefined and null values in javascript when programming()
Pingback: Wrapper objects in Javascript and access properties of literals()