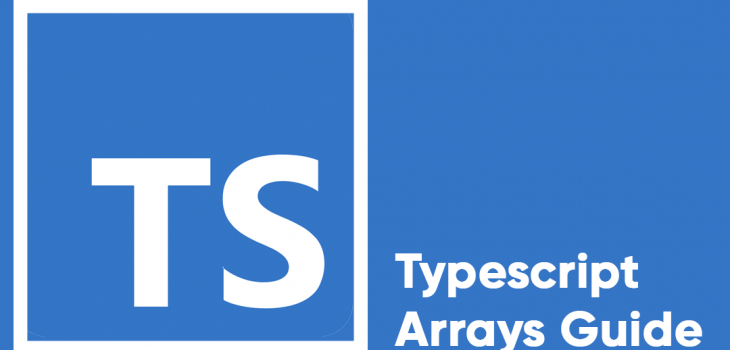
How to use Typescript with arrays
Array is used to store a sequence of elements as references. When declaring typescript array, the type of the elements should be defined in its syntax.
1. Initialize Array
Create Array with the type
const stringArray1:string[] = ['a', 'b']; const stringArray2:Array = ['a', 'b']; const numberArray1:number[] = [1, 2]; const numberArray2: Array = [1, 2];
Both of the above declarations are similar. One follows T<U>
generic signature and other uses primitive types.
2. Declare and assign values
First, we are going to initialize the array and then assign the value.
let array1: string[]; array1 = ['apple', 'banana']; let array2:Array<string>; array2 = ['apple', 'banana'];
Note that you cannot assign different values to the declared types. It will give a linting error from the type checker.
let errorArray:Array<string>; errorArray = [1, '12']; // Type error
3. Use multiple data types in same array without order
You can use two or more types of data in a single array without a specific order as follows
let array1:Array = [1, '12']; let array2:(string | number)[] = [2, 'foo']; let array3:Array; array3 = [1, '12'];
4. Create array with types or tuples
This is a way to create an array specific with types along with the position of the element
let array1:[number, string] = [1, '2']; let array2:[number, string] = ['doo', 'foo']; // Error
One of the common example is React hooks you find in functional components
type SetStateType = [string, (param: string) => void]; const useState = (value:string): SetStateType => { let state = value; return [state, (param: string) => { state = param; } ]; }; let [value, setValue]: SetStateType = useState('string'); // this is not the exact implementation. Just a demonstration
5. Usage with generics
You can use an array with generics as in the following example.
interface Person {
name: string;
age: number;
}
let personArray1:Person[] = [{name: 'John', age: 12}];
let personArray2:Array = [{name: 'Doe', age: 22}];
6. Examples
Following are some examples with array prototype functions.
interface Person { name: string; age: number; } let people:Person[] = [{name: 'John', age: 12}]; people.push({name: 'Doe', age: 33}); people.splice(0, 1, {name: 'Foo', age: 15}); console.log(people[0].name); // "Foo" const elderPeople:Array<Person> = people.filter(p => p.age > 13); if (elderPeople.length > 0) { const elderNames: string[] = elderPeople.map(p => p.name); console.log('Elder guys: ', elderNames.join(', ')); // "Elder guys: ", "Foo, Doe" } const elderCircle = elderPeople.concat({name: 'Pony', age: 1}); console.log(elderCircle[2].name); // "Pony"