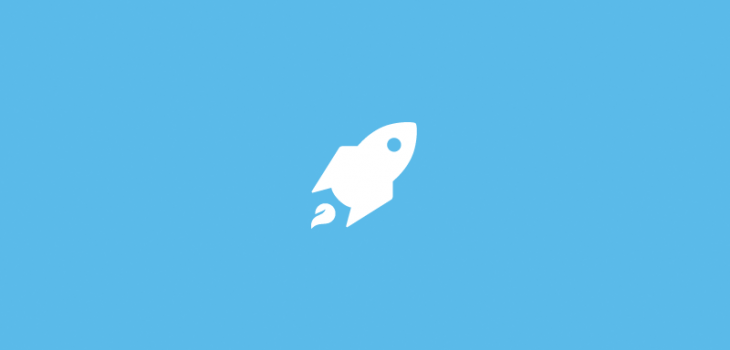
Servlet File Upload
HOW TO UPLOAD FILES AND EXTRACT OTHER DATA FROM A POST SUBMISSION IN A SERVLET
In working with java EE, the submission of data from a JSP or a Servlet is basically handled by Struts or Spring frameworks. But for programmers, who may have an interest in the things in a POST Request, will find some use in this. Especially, if you intend to build a separate framework for any web application.
First of all, make a JSP page with a form consisting two input fields and a file field.
<form action="UploadInfo" method="POST" enctype="multipart/form-data"> <label>NAME</label><input type="text" name="cust_name" /><br /> <label>TELEPHONE</label><input type="text" name="cust_tel" /><br /> <label>PICTURE</label><input type="file" name="cust_pic" /><br /> <input type="submit" value="ADD" /><br /> </form>
Please don’t bother CSS and other decorations, coz I haven’t used any. Now I’m going to code the servlet for the upload action
The code will go inside protected void doPost(HttpServletRequest request, HttpServletResponse response) method of the UploadInfo Servlet. We have to be certain whether the request has a file to upload in the first place. If not, there will be no confusion in retrieving the String data from the POST request.
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { response.setContentType("text/html;charset=ISO-8859-1"); PrintWriter out = response.getWriter(); try { String contentType = request.getContentType(); String imageLocation =""; String imageName=""; String name=""; String tel=""; if ((contentType != null) && (contentType.indexOf("multipart/form-data") >= 0)) { DataInputStream in = new DataInputStream(request.getInputStream()); int formDataLength = request.getContentLength(); byte dataBytes[] = new byte[formDataLength]; int byteRead = 0; int totalBytesRead = 0; while (totalBytesRead < formDataLength) { byteRead = in.read(dataBytes, totalBytesRead, formDataLength); totalBytesRead += byteRead; } String file = new String(dataBytes); String saveFile = file.substring(file.indexOf("filename=\"") + 10); saveFile = saveFile.substring(0, saveFile.indexOf("\n")); saveFile = saveFile.substring(saveFile.lastIndexOf("\\") + 1, saveFile.indexOf("\"")); int lastIndex = contentType.lastIndexOf("="); String boundary = contentType.substring(lastIndex + 1, contentType.length()); int pos; pos = file.indexOf("filename=\""); pos = file.indexOf("\n", pos) + 1; pos = file.indexOf("\n", pos) + 1; pos = file.indexOf("\n", pos) + 1; imageName = "hello" + saveFile.substring(saveFile.length() - 4); imageLocation = getServletContext().getRealPath("")+ "\\images\\" + imageName; int boundaryLocation = file.indexOf(boundary, pos) - 4; int startPos = ((file.substring(0, pos)).getBytes()).length; int endPos = ((file.substring(0, boundaryLocation)).getBytes()).length; File f = new File(imageLocation); FileOutputStream fileOut = new FileOutputStream(f); fileOut.write(dataBytes, startPos, (endPos - startPos)); fileOut.flush(); fileOut.close(); /** * Retrieves other fields in the POST request */ name=retrieveName(file, "cust_name"); tel=retrieveName(file, "cust_tel"); } out.println("<html>"); out.println("<head>"); out.println("<title>Servlet UploadInfo</title>"); out.println("</head>"); out.println("<body>"); out.println("<p>Name : " + name + "<br> Tel : " + tel + "</p>"); out.println("<img src=\"" +imageName + "\" />"); out.println("</body>"); out.println("</html>"); } finally { out.close(); } } private String retrieveName(String file, String name) { String[] split = file.split("\"" + name + "\""); if (split.length >= 2) { return split[1].toString().substring(0, split[1].indexOf("------")).trim(); } else { return null; } }
String contentType = request.getContentType(); if ((contentType != null) && (contentType.indexOf("multipart/form-data") >= 0)) { }
This will check whether request has any content type and it has some file format in it. Now, you can code DataStream works inside those braces.
DataInputStream in = new DataInputStream(request.getInputStream()); int formDataLength = request.getContentLength(); byte dataBytes[] = new byte[formDataLength]; int byteRead = 0; int totalBytesRead = 0; while (totalBytesRead < formDataLength) { byteRead = in.read(dataBytes, totalBytesRead, formDataLength); totalBytesRead += byteRead; } String file = new String(dataBytes);
Above code is to take the stream to a String.
String saveFile = file.substring(file.indexOf("filename=\"") + 10); saveFile = saveFile.substring(0, saveFile.indexOf("\n")); saveFile = saveFile.substring(saveFile.lastIndexOf("\\") + 1, saveFile.indexOf("\""));
Above is to get the file name.
int lastIndex = contentType.lastIndexOf("="); String boundary = contentType.substring(lastIndex + 1, contentType.length()); int pos; pos = file.indexOf("filename=\""); pos = file.indexOf("\n", pos) + 1; pos = file.indexOf("\n", pos) + 1; pos = file.indexOf("\n", pos) + 1; String imageName = "hello" + saveFile.substring(saveFile.length() - 4); String imageLocation = getServletContext().getRealPath("") + "\\" + imageName; int boundaryLocation = file.indexOf(boundary, pos) - 4; int startPos = ((file.substring(0, pos)).getBytes()).length; int endPos = ((file.substring(0, boundaryLocation)).getBytes()).length;
Above is to get the position of the image data correctly. You can understand the above code if you JUST PRINT the file String in the response.
File f = new File(imageLocation); FileOutputStream fileOut = new FileOutputStream(f); fileOut.write(dataBytes, startPos, (endPos - startPos)); fileOut.flush(); fileOut.close();
Above is to get the position of the image data correctly. You can understand the above code if you JUST PRINT the file String in the response.
private String retrieveName(String file, String name) { String[] split = file.split("\"" + name + "\""); if (split.length >= 2) { return split[1].toString().substring(0, split[1].indexOf("------")).trim(); } else { return null; } }
Above method is to retrieve cust_name and the cust_tel field from the POST submission as getParameter(String name) won’t work.