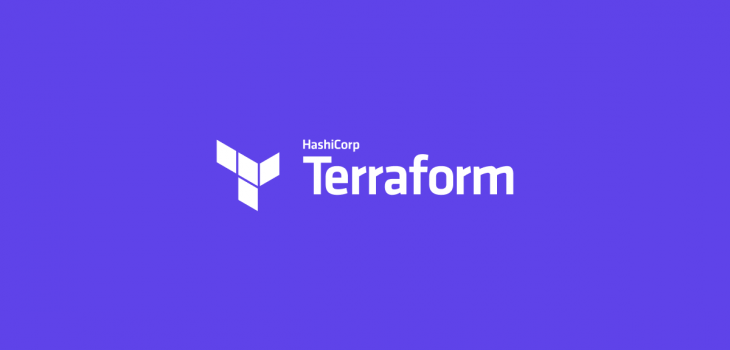
How to read AWS credential profiles with Terraform
We often use AWS credentials file when using AWS CLI to switch between different accounts. You can also read AWS credential profiles with Terraform and use it to work with the terraform CLI commands. For that the cleanest method is to use the provider in .tf
file to define the config in your aws provider.
In your main.tf
file where the aws provider is defined, you can provide the AWS credentials file you want to use.
provider "aws" {
region = "us-east-1" # region of the deployment
profile = "test" # name of the profile in credentials file
}
The credentials file should look like following. The default location of the credential file is ~/.aws/credentials
aws_access_key_id=****
aws_secret_access_key=********
region=**-****-*
[test]
aws_access_key_id=****
aws_secret_access_key=********
region=**-****-*
[production-app]
aws_access_key_id=****
aws_secret_access_key=********
region=**-****-*
Profile name is what we have inside the square brackets. For example in the above credentials file we have default, test, production-app
as profiles. Each profile will contain the access_key
and the secret_key
to authenticate the CLI.
If you have a seperate location for the aws credentials file you can provide following as the path of the credential file.
"aws" {
region = "us-east-1" # region of the deployment
profile = "test" # name of the profile in credentials file
shared_credentials_file = "/home/users/test/aws/credentials" # path of the credentials file
}
Now you can call terraform apply
and it will choose the relevant profile from the credentials file to apply.
You can check more details in the official documentation for AWS Terraform to see what can be configured in the providers blog.