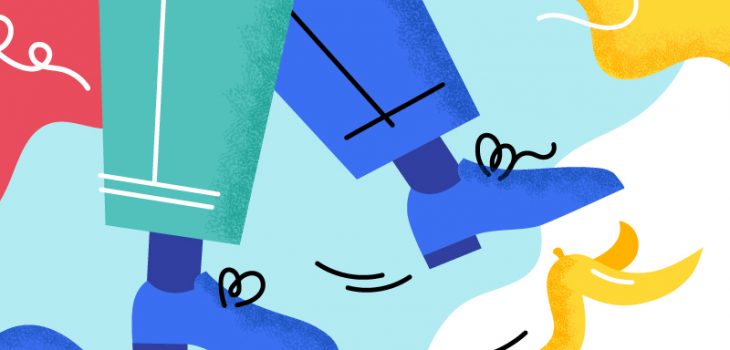
Query definition in GraphQL and frequent errors with Apollo…
Query definition in GraphQL is will be something you know now but not mastered. If you were going through the pages to troubleshoot frequent errors with Apollo Client which makes no sense, this post might help. First, the reason to create this post is,
- Most of the error logs are not descriptive and makes no clue on where the real error is.
- There can be so many different mistakes in coding the queries and difficulty in keeping on track of it.
And most importantly if I get into a trouble again, I can refer this 😉
Basics of Query Definition in GraphQL
The basic query has following structural reference in its queries.
query Operation($variable: Type! = DefaultVariable, $directive) { alias: listEvents(argument: $variable) { field1 field2 field3 fieldList @include(if: $directive) { field4 } ...fragmentFields } } fragment fragmentFields on Type { fragmentField1 fragmentField2 }
- fields: Attributes which are returned as response from an object representation
- arguments: Values which determines the conditions that the data is returned
- aliases: Remap/Categorize the returned data on a provided condition
- directive: Conditions to include or exclude fields
- fragments: Get fragment of data from same type definition
Note that above is a simple representation and this can be supplemented with more complex or matured APIs. You can refer these at https://graphql.org/learn/queries/#directives
Query mistakes when coding GraphQL
If you have seen following frequent errors with Apollo Client when trying to subscribe you can check it and validate whether these mistakes are with it.
1. Cannot read property ‘subscription’ of undefined
What can you understand by this error message? First impression you get is the subscription is not initialized. But this is not the case here. This happens when the query parameters are missing or not provided properly.
For example take this query.
const SubscribeToPosition = gql`subscription OnUpdatePosition($userId: String!) { onUpdatePosition(userId: $userId) { placeId position lastUpdated } }`
To subscribe to the position updates we need to provide the userId
as a parameter when client is subscribing to the event.
apolloClient.hydrated().then((client) => { client.subscribe({ query: SubscribeToPosition, variables: { placeId, }}) .subscribe({ next: (data) => { dispatch(Actions.position.onUpdatePosition(data)); }, //complete: complete action, //error: error action, }); }); });
If your attributes in the variables sent with the client are not similar to the variables in the query, this error will be occurring. In this example its placeId. I really hope this error message will be changed in future releases.
(bdw, checkout this blog if you need to setup GraphQL: https://sandny.com/2018/09/24/appsync-graphql-amplify-iam/)
2. Subscriptions are not registered and no events are received
This is a vague problem and it can occur due to several reasons.
- The subscription query, syntax has errors.
These errors are mostly throws an error. Or does return false data. You can always code these subscriptions and check with AppSync queries whether its working or not. Follow the query syntax and code the subscription. Then test whether its working with the AppSync query browser.
If the subscriptions are activated when mutating the data, so will it with Apollo Client. So that will be the kind of 1st step of diagnosing subscriptions
- The subscription fields are not defined in the mutation query
One of the common mistake is that you face when creating subscriptions is this one. The reason why you will loose your hair count is, it doesn’t have any clue! If you have a mutation and you dont want to receive any data, you will create a mutation query like this.
mutation CreateUser { createUser(input: { userId: "uuid-123", jobId: "uuid-jobId", firstName: "Tony", lastName: "Stark", lastUpdated: "74640371801", }) { userId } } subscription OnCreateUser($jobId: String!) { createUser(jobId: $jobId) { userId jobId firstName lastName lastUpdated } }`;
This subscription wont be able to receive any data since the mutation doesn’t have the return fields. This is specific factor for AppSync
- Not authorized or credential errors
- Other Errors
Following will be some errors that you will face when implementing your code with AWS AppSync. I will update it frequently with the errors
- The provided key element does not match the schema:
Not Providing the whole key structure to the query. Probably you have missed out the sort key.
(PS: I will be updating the list as I find errors)