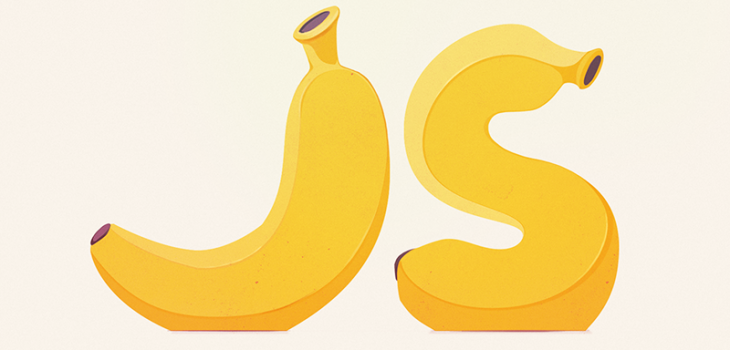
Higher order component wrappers using React.js and reusable components.
Recently I happened to create a middle panel where different types of components could be rendered on top it. So the idea is, the panel should be a <div> wrapper around the middle content. For this kind of situation, higher order functions with components could come in handy to use.
Let’s say our middle content is order detail panel. First I had two buttons that displayed exactly same it was an expandable button. So I first created a button which could take the text and an action to be dispatched from when the onClick event fires.
I use stateless functions as much as I can to create components. And the state is managed with Redux.js. So the expandable button is as follows:
const DetailExpandableButton = (props) => { return ( <div className="expandable-button"> <div className="eb-text">{props.text}</div> <div className="eb-expand-button" onClick={props.action}>+</div> </div> ); }
This was used in order detail component as follows:
const CustomerDetails = ({showCustomer, showOrderType}) => { return ( <div className="customer-details"> <div className="add">{DetailExpandableButton({text: "Customer", action: showCustomer})}</div> <div className="add">{DetailExpandableButton({text: "Order Type", action: showOrderType})}</div> </div> ) }
And the order detail panel wrapped around with middle content. Note that the actions defined here are redux dispatch functions which are defined in another context. I have used them directly here to render other components.
export const CustomerDetailsMiddlePane = MiddlePane(CustomerDetails({ showCustomer: CustomerActions.showCustomerDetails, showOrderType OrderActions.showOrderTypes, }));
The middle content is a wrapper for the customer details and it will render the component passed to the middle content at runtime.
export const MiddlePane = (Content) => (props) => ( <div className="middle-pane"> <Content {...props}/> </div> );
So that’s it. Now I have a middle pane which I can be reused to render some other components. And expandable button which could be literally used anywhere with the text and the action. It’s easier and much cleaner as I’m using only stateless functional components. There could be a need for stateful components (to manage tap events etc.) but its always easier to use more stateless ones.