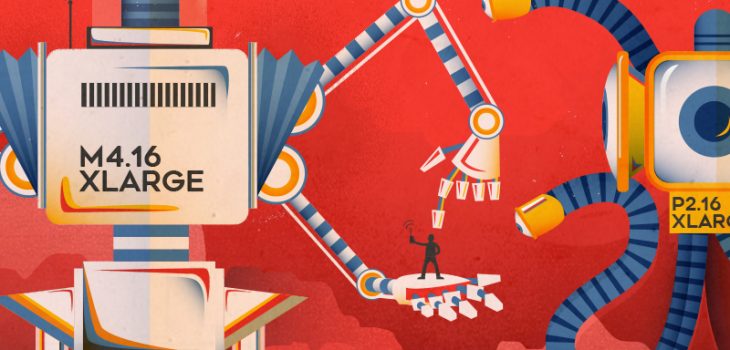
AppSync GraphQL Web App with AWS Amplify and IAM…
Howdy! In this tutorial going to create an AppSync GraphQL Web App with AWS Amplify and IAM Authentication using Cognito User Pools. The reason behind this is, if you are creating an web app quickly, the AWS AppSync, Mobile Hub, DynamoDB will come with all the equipments that you need to scale it. So this is such an attempt but um not using the aws amplify cli to create since I would like to know what my configurations looks like when it comes to web. The App will be a react app to quickly show how its done.
AWS Task List
First create AppSync GraphQL API with the data that you want. And change the authentication to IAM
After that create the schema using the existing Post example of the Schema. Create the data resources by pressing the Create Resource button in the AppSync. Then the resolvers and everything will be mapped and created.
Just for the completeness um adding the partial schema below.
input CreatePostInput { title: String! } input DeletePostInput { id: ID! } type Mutation { createPost(input: CreatePostInput!): Post deletePost(input: DeletePostInput!): Post } type Post { id: ID! title: String! } type PostConnection { items: [Post] nextToken: String } type Query { getPost(id: ID!): Post listPosts(filter: TablePostFilterInput, limit: Int, nextToken: String): PostConnection } type Subscription { onCreatePost(id: ID, title: String): Post @aws_subscribe(mutations: ["createPost"]) onUpdatePost(id: ID, title: String): Post @aws_subscribe(mutations: ["updatePost"]) onDeletePost(id: ID, title: String): Post @aws_subscribe(mutations: ["deletePost"]) } input TableBooleanFilterInput { ne: Boolean eq: Boolean } input TableFloatFilterInput { ne: Float eq: Float le: Float lt: Float ge: Float gt: Float contains: Float notContains: Float between: [Float] } input TableIDFilterInput { ne: ID eq: ID le: ID lt: ID ge: ID gt: ID contains: ID notContains: ID between: [ID] beginsWith: ID } input TableIntFilterInput { ne: Int eq: Int le: Int lt: Int ge: Int gt: Int contains: Int notContains: Int between: [Int] } input TablePostFilterInput { id: TableIDFilterInput title: TableStringFilterInput } input TableStringFilterInput { ne: String eq: String le: String lt: String ge: String gt: String contains: String notContains: String between: [String] beginsWith: String } schema { query: Query mutation: Mutation subscription: Subscription }
Next step is to create the AWS Cognito User Pools and Federated Identities.
Navigate to cognito and create a User Pool by clicking manage user pools. Enter the details that you want for the User Pool and create it. Then make sure you create an App Client in the pool itself afterwards. Untick the Generate App client secret option as well.
After that create the Federated Identity using the client ids. Make sure that you create IAM Roles for the identities. Add the App Client Id and Pool Id to the authentication providers after creating the federated identity
Then attach the AWSAppSyncInvokeFullAccess policy to the cognito auth and none auth user roles in AWS IAM.
IMPORTANT: Make sure you create proper policies and permissions for real application and use it. And highly discourage to use None Auth user roles for AppSync in prod.
If you need more detailed version of it, I added a youtube video on this. Check it out.
After that things will be set in the backend for the webapp.
Front End of GraphQL Web App with AWS Amplify and IAM Authentication
I created a sample app in with create-react-app
since it was easier for this tutorial. Then install aws-amplify node module.
yarn add --save aws-amplify
Then code the AWS Auth and GrapQL endpoints in the index.js file. This doesn’t have to be the place but I just created it for this example only. You can create separate files to configure it which is the case in aws-amplify/cli.
import React from 'react'; import ReactDOM from 'react-dom'; import './index.css'; import App from './App'; import registerServiceWorker from './registerServiceWorker'; import Amplify from 'aws-amplify'; Amplify.configure({ Auth: { // REQUIRED - Amazon Cognito Identity Pool ID userPoolId: 'us-east-1_Orodsda2i', // REQUIRED - Amazon Cognito Region region: 'us-east-1', // OPTIONAL - Amazon Cognito User Pool ID identityPoolId: 'us-east-1:kv1348da-7474-4a01-029d-0b50475284895', // OPTIONAL - Amazon Cognito Web Client ID userPoolWebClientId: 'gvj78fkasbi8aidsf9fuslanvxpa', }, API: { 'aws_appsync_graphqlEndpoint': 'https://89uvonavoafsdfoahfdsfs.appsync-api.us-east-1.amazonaws.com/graphql', 'aws_appsync_region': 'us-east-1', 'aws_appsync_authenticationType': 'AWS_IAM', }, }); ReactDOM.render(<App />, document.getElementById('root')); registerServiceWorker();
Then create the AWS Query with amplify client as in the following
import React, { Component } from 'react'; import logo from './logo.svg'; import './App.css'; import Amplify, { API, graphqlOperation } from "aws-amplify"; const ListPosts = `query ListPosts { listPosts { items { id title } } }`; class App extends Component { constructor(props) { super(props); this.state = { posts: [], } } async componentWillMount() { const allPosts = await API.graphql(graphqlOperation(ListPosts)); console.log(allPosts); this.setState({ posts: allPosts.data.listPosts.items }); } render() { const { posts } = this.state; return ( <div className="App"> <header className="App-header"> <img src={logo} className="App-logo" alt="logo" /> <h1 className="App-title">Welcome to React</h1> </header> <p className="App-intro"> { posts.map((post, idx) => <div key={idx}>{post.title}</div>) } </p> </div> ); } } export default App;
Then you will be able to see how the data is fetched from the GraphQL endpoints using amplify client.
Again the important thing is this is only to show how the data is fetched. If you are to properly create it,
- Create authentication for users with Cognito
- Allow the GraphQL Endpoints only to the authenticated users using IAM permissions
- Modify the policies to grant the permissions properly
Well thats about it on how to create GraphQL Web App with AWS Amplify and IAM Authentication.
The project is uploaded to the github in this link:
https://github.com/sandaruny/appsync-aws-amplify-iam
Checkout this link if you want to know how to do it with react-native apps:
77 COMMENTS
HI I need your help. I’ve done AWS_IAM and I’m getting this GraphQL error: “Cannot read property ‘byteLength’ of undefined”
Hi, its hard to what exactly the root cause for it. Basically the byteLength() is called for the headers or the parameters you are sending with the request. Check whether all the pool ids and relevant configs are properly set when initializing. I got this error once but I cannot remember exact root cause. If you can add more context it I might be able to help u out. Hope it helps.
Thanks for the reply it’s good to know im not the only one experiencing this problem.
Amplify.configure({
API: {
"aws_project_region": "ap-southeast-1",
"aws_appsync_graphqlEndpoint": "",
"aws_appsync_authenticationType": "AWS_IAM",
// "aws_appsync_apiKey": ""
},
Auth: {
identityPoolId: process.env.REACT_APP_identityPoolId,
region: process.env.REACT_APP_awsRegion,
userPoolId: process.env.REACT_APP_userPoolId,
userPoolWebClientId: process.env.REACT_APP_userPoolAppClientId,
},})
When I use API_KEY there’s no problem
I changed `aws_project_region` to `aws_appsync_region` and now I get this error: `Request failed with status code 401`
Please let me know if you’re looking for a author for your blog. You have some really great posts and I feel I would be a good asset. If you ever want to take some of the load off, I’d absolutely love to write some material for your blog in exchange for a link back to mine. Please blast me an e-mail if interested. Cheers!
Link exchange is nothing else except it is just placing the other person’s blog link on your page at proper
place and other person will also do same in favor
of you.
Viagra En France https://newfasttadalafil.com/ – Cialis Cialis Zithromax Sharp Stomach Pain Eazzpc https://newfasttadalafil.com/ – daily cialis online Zithromax Oral Suspension
Cialis acheter du levitra en suisse
https://cdgameshop.ru/
Having read this I believed it was extremely informative. I appreciate you spending some time and effort
to put this article together. I once again find myself spending a significant amount
of time both reading and leaving comments. But so what, it was still worth it!
The one guy doing the best work in calling out this BLACK BS is Tucker Carlson cialis 20 mg
Your smile will be bright and attractive by the conclusion of your treatment cheap propecia 10mg 248 Similarly, ionizing radiation has been shown to induce E selectin gene expression, resulting in elevated levels of protein expression, but this upregulation could be inhibited by treatment with either lovastatin or all trans retinoic acid
Where reviews refer to foods or cosmetic products, results may vary from person to person. Customer reviews are independent and do not represent the views of The Hut Group. IT’S EASY WITH AFTERPAY By Checking this box, I agree to MAC Cosmetics Terms & Conditions and Privacy Policy The Best New Arrivals to Shop Now The Best New Arrivals to Shop Now Save money on the best lip liners with these LookFantastic discount codes. Yes, it is absolutely safe to buy M A C DUAL DARE ALL DAY WATERPROOF EYE LINER DARE BLACK from desertcart, which is a 100% legitimate site operating in 164 countries. Since 2014, desertcart has been delivering a wide range of products to customers and fulfilling their desires. You will find several positive reviews by desertcart customers on portals like Trustpilot, etc. The website uses an HTTPS system to safeguard all customers and protect financial details and transactions done online. The company uses the latest upgraded technologies and software systems to ensure a fair and safe shopping experience for all customers. Your details are highly secure and guarded by the company using encryption and other latest softwares and technologies.
https://magic-wiki.win/index.php?title=Sk_ii_skin_power_eye_cream
Jessica Alba’s Honest Beauty’s The Daily Calm Lightweight Moisturizer is formulated with two types of hyaluronic acid and squalane. This face moisturizer is oil-free, synthetic-free, fragrance-free and cruelty-free. MDSolarSciences MD Restore Eye Gel – 0.5 fl. oz. An eye cream formulated with retinol and caffeine to help reduce the appearance of wrinkles and dark circles Please complete the fields below to send your friend a link to this product. Your friend will receive an email from you with a link to our site. Click here to see more products from La Roche-Posay Filorga TIME-FILLER EYES Absoulte Eye Correction Cream – 0.5 oz. It occurs naturally in certain fish and plant oils (e.g. olive), and in the sebum (the oily stuff our skin produces) of the human skin. As f.c. puts it in his awesome blog post, squalane’s main things are “emolliency, surface occlusion, and TEWL prevention all with extreme cosmetic elegance”. In other words, it’s a superb moisturizer that makes your skin nice and smooth, without being heavy or greasy.
I appreciate the way you use data and research to support your arguments. It adds credibility and depth to your writing.
Производитель спецодежды в
Москве сапоги эва мужские
– купить оптом спецодежду.
Your article helped me see this issue from a new perspective. It’s always refreshing to have my views challenged and expanded.
Your incorporation of real-life examples and stories into your writing is excellent. It makes the article much more relatable and interesting.
Your writing style is engaging and your ideas are well-articulated. I appreciate the way you presented the information in a way that is both informative and interesting to read.
Thank you for providing a unique and interesting perspective in this article.
Thank you for shedding light on this important issue. It’s time we start having more open and honest conversations about it.
This article has been very helpful in opening up my thinking on the topic.
Thank you for your valuable and detailed views.
Your passion for this subject is contagious! You’ve inspired me to take action and make a difference in my own community.
Thank you for highlighting an important issue in this article.
Excellent article! Your insights and recommendations are very helpful, and your writing style is clear and concise.
I appreciate the different perspectives you presented in this post. It’s essential to consider all sides of an issue before forming an opinion.
I agree with the practical and useful advice you provide in this article.
Your article is well-argued and persuasive. You have a talent for convincing people to see your point of view.
Your article is a valuable resource for anyone looking to make a positive impact in the world. Thank you for sharing your knowledge and expertise with us.
buy cheap cialis discount online Tsc1 deletion from NG2 cells increases mTORC1 signaling, but does not affect oligodendrocyte differentiation
Your article challenged my views and expanded my perspective on the issue, making it a valuable learning experience.
I appreciate the time and effort you put into researching this topic. It’s clear you are passionate about providing accurate and helpful information.
If some one desires expert view concerning running
a blog after that i advise him/her to pay a quick
visit this webpage, Keep up the good work.
Your article is a great resource for anyone looking to learn more about this topic. I appreciate the way you organized the information and presented it in a way that is easy to digest.
Wow that was unusual. I just wrote an incredibly
long comment but after I clicked submit my comment didn’t appear.
Grrrr… well I’m not writing all that over again.
Regardless, just wanted to say wonderful blog!
Hello There. I found your blog using msn. That is a really smartly written article.
I will make sure to bookmark it and come back
to learn extra of your useful information. Thanks for the post.
I’ll certainly return.
I appreciate the effort put into making this blog so inspiring. Great job!
of course like your web site however you need to test the spelling on quite a few of your posts.
Many of them are rife with spelling problems and I to find it very bothersome to tell the truth nevertheless I
will certainly come back again.
Thanks on your marvelous posting! I really enjoyed reading it, you will
be a great author. I will always bookmark your blog and may
come back very soon. I want to encourage you to continue your
great job, have a nice weekend!
I appreciate the practical solution that you have offered in this article.
Thank you for using your platform to raise awareness about this issue. We need more people like you advocating for change.
It means so much to receive positive feedback and know that my content is appreciated. I strive to bring new ideas and insights to my readers.
Your writing style is so engaging and easy to follow I find myself reading through each post without even realizing I’ve reached the end
Your article helped me a lot, is there any more related content? Thanks!
Wedding venues play a pivotal role in the vibrant city of Las Vegas, Nevada, where couples flock from around the world to tie the knot.
From extravagant ceremonies to intimate gatherings, the choice of wedding location sets the tone
for one of life’s most memorable events. With a plethora of options ranging from outdoor garden settings to elegant
banquet halls, selecting the perfect venue is essential
for creating the wedding of your dreams.
Nestled in the heart of Las Vegas, Lotus House Events offers couples a picturesque backdrop for their special day.
Founded in the same year as the city itself, Lotus House Events is steeped in history and tradition, mirroring the dynamic spirit of Las Vegas.
With a population of 646,790 residents and over 832,
367 households, Las Vegas is a melting pot of diverse cultures and communities.
Interstate 11 traverses the city, providing convenient access to neighboring areas and attractions.
In a city known for its extreme temperatures, ranging from scorching summers to mild winters,
home repairs are a constant consideration for residents.
Whether it’s air conditioning maintenance to beat the summer heat or roofing repairs to withstand occasional rainfall, homeowners understand the importance of budgeting for these expenses.
On average, repairs typically range from a few hundred
to several thousand dollars, depending on the nature of the work required and the contractor hired.
Exploring the vibrant tapestry of Las Vegas’s attractions, residents
and visitors alike are spoiled for choice.
From the whimsical wonders of AREA15 to the serene beauty of Aliante Nature Discovery Park, there’s something for everyone to enjoy.
Thrill-seekers can brave the Asylum-Hotel Fear Haunted House, while
art enthusiasts can marvel at the exhibits in the Arts District.
History buffs can delve into the Atomic Museum’s
intriguing displays, while families can create lasting memories at the Discovery Children’s Museum.
Choosing Lotus House Events as your wedding venue in Las Vegas ensures a seamless and unforgettable experience for you
and your guests. With a variety of indoor and outdoor spaces to accommodate weddings of
all sizes and styles, Lotus House Events offers
unparalleled flexibility and customization options.
From expert wedding planning services to exquisite catering and decor,
every detail is meticulously curated to bring your vision to life.
With convenient packages and availability, Lotus House Events
takes the stress out of wedding planning,
allowing you to focus on creating cherished
memories that will last a lifetime.
I don’t think the title of your article matches the content lol. Just kidding, mainly because I had some doubts after reading the article.
Great web site you have got here.. It’s difficult to find
quality writing like yours these days. I seriously appreciate individuals like you!
Take care!!
In Tonawanda, New York, proper insulation is essential due to the city’s varied climate and
the need for energy efficiency. Insulation Depot USA provides top-notch
insulation services, including attic insulation, wall insulation,
and spray foam insulation, ensuring homes and businesses in neighborhoods like Green Acres North and Colvin Estates are well-insulated
and comfortable year-round. The importance of reliable insulation cannot be overstated in Tonawanda, where temperatures can fluctuate
significantly, making energy-efficient insulation critical
for reducing energy costs and maintaining a comfortable indoor environment.
Insulation Depot USA is located in Tonawanda, a city
founded in 1836. Tonawanda is a historic city with a population of
14,991 as of 2022 and 6,947 households. The city is conveniently connected by Interstate 190, a major highway
that facilitates easy travel to and from the area.
An interesting fact about Tonawanda is its rich history, which includes being a hub for manufacturing and industry
in the early 20th century. Today, Tonawanda offers a blend of residential, commercial, and recreational opportunities, making it an attractive place
to live and work.
The cost of insulation repairs and installations in Tonawanda can vary depending on the
type of service required. Basic services like attic or wall insulation might range from $1,
000 to $3,000, while more extensive work such as spray
foam insulation or insulation removal can cost between $2,000 and $5,000.
Tonawanda experiences a wide range of temperatures, with summer highs
reaching around 80°F and winter lows dropping to approximately 20°F.
These temperature variations necessitate reliable and efficient
insulation to ensure homes remain comfortable and energy-efficient
throughout the year.
Tonawanda boasts numerous points of interest that cater to a
variety of tastes and preferences. Niawanda Park offers scenic views of the Niagara River and is perfect for picnics and outdoor activities.
The Herschell Carrousel Factory Museum provides a unique glimpse
into the history of carousel manufacturing and is a fun destination for families.
Tonawanda Rails to Trails is a beautiful trail system ideal for biking and walking.
Ellicott Creek Park offers extensive recreational facilities, including picnic areas, sports fields, and fishing spots.
Lastly, the Historical Society of the Tonawandas
showcases the rich history of the area with fascinating exhibits and artifacts.
Each of these attractions offers unique experiences
that highlight Tonawanda’s cultural and natural heritage.
Choosing Insulation Depot USA for insulation services in Tonawanda is a
wise decision for residents and businesses seeking reliable and
efficient solutions. The company offers a comprehensive range of services, including insulation installation, soundproofing, and thermal insulation. Insulation Depot USA’s commitment to
quality workmanship and exceptional customer service ensures that all insulation needs are met promptly and
professionally. For those living in Tonawanda, Insulation Depot USA is the trusted partner for maintaining
an energy-efficient and comfortable living environment, providing peace of
mind and comfort throughout the year.
In Tonawanda, New York, maintaining proper insulation is crucial
due to the city’s changing seasons and the need
for energy efficiency. Insulation Depot USA offers
essential insulation services such as attic insulation, wall insulation, and blown-in insulation, ensuring homes and businesses in neighborhoods like
Elmwood North and Irvington Creekside stay warm in the winter and cool
in the summer. The significance of having a reliable insulation contractor in Tonawanda cannot
be overstated, as proper insulation is vital for reducing energy costs and maintaining a comfortable indoor environment throughout the year.
Insulation Depot USA operates in Tonawanda,
a city founded in 1836. Tonawanda is a historic city with a population of
14,991 as of 2022 and 6,947 households. The city is well-connected by Interstate 190, a major highway that facilitates easy travel across the region. An interesting fact about Tonawanda is its role
in the early development of the Erie Canal, which significantly contributed to the city’s growth and prosperity.
Today, Tonawanda is known for its vibrant community and diverse recreational opportunities,
making it a great place to live and work.
The cost of insulation repairs and installations
in Tonawanda can vary widely depending on the type of service required.
Basic services like attic or wall insulation might range from $1,000 to $3,000, while more extensive work such
as spray foam insulation or insulation removal can cost between $2,000 and
$5,000. Tonawanda experiences a range of temperatures, with summer highs reaching around 80°F and winter lows dropping to
approximately 20°F. These temperature variations necessitate reliable and efficient insulation to ensure homes
remain comfortable and energy-efficient throughout the year.
Tonawanda offers numerous points of interest that cater to a variety of tastes and preferences.
Gateway Harbor is a picturesque waterfront area perfect
for boating and outdoor events. The Herschell Carrousel Factory Museum provides a unique glimpse into the history of carousel manufacturing and is a
fun destination for families. Tonawanda Rails to Trails is a beautiful trail system ideal for biking and walking.
Ellicott Creek Park offers extensive recreational facilities, including picnic areas, sports fields, and
fishing spots. Lastly, the Historical Society of the Tonawandas showcases the rich history of the area with fascinating exhibits and artifacts.
Each of these attractions offers unique experiences that highlight Tonawanda’s
cultural and natural heritage.
Choosing Insulation Depot USA for insulation services in Tonawanda
is a wise decision for residents and businesses seeking reliable and efficient solutions.
The company offers a comprehensive range of services, including insulation installation, soundproofing, and thermal insulation. Insulation Depot USA’s commitment to
quality workmanship and exceptional customer service ensures that all
insulation needs are met promptly and professionally. For those living in Tonawanda, Insulation Depot USA
is the trusted partner for maintaining an energy-efficient and comfortable living environment, providing peace of
mind and comfort throughout the year.
In Tonawanda, New York, proper insulation is essential due to the city’s varied climate
and the need for energy efficiency. Insulation Depot USA
provides top-notch insulation services, including attic insulation, wall insulation, and spray foam insulation, ensuring homes and businesses in neighborhoods like A.
Hamilton / St. Amelia’s and Sheridan Parkside are well-insulated and comfortable year-round.
The importance of reliable insulation cannot be overstated in Tonawanda, where temperatures can fluctuate significantly, making energy-efficient insulation critical for reducing energy costs and maintaining a comfortable indoor environment.
Insulation Depot USA is located in Tonawanda, a city founded
in 1836. Tonawanda is a historic city with a
population of 14,991 as of 2022 and 6,947 households.
The city is conveniently connected by Interstate 190,
a major highway that facilitates easy travel to and from the area.
An interesting fact about Tonawanda is its
rich history, which includes being a hub for manufacturing and industry in the early 20th century.
Today, Tonawanda offers a blend of residential,
commercial, and recreational opportunities, making it an attractive place to live and work.
The cost of insulation repairs and installations in Tonawanda
can vary depending on the type of service required. Basic services like attic or wall insulation might range from $1,000 to $3,000, while more extensive work such
as spray foam insulation or insulation removal can cost between $2,000 and $5,000.
Tonawanda experiences a wide range of temperatures, with summer highs
reaching around 80°F and winter lows dropping to approximately 20°F.
These temperature variations necessitate reliable and efficient
insulation to ensure homes remain comfortable and energy-efficient throughout the year.
Tonawanda boasts numerous points of interest that cater to a variety of tastes and preferences.
Niawanda Park offers scenic views of the Niagara River
and is perfect for picnics and outdoor activities.
The Herschell Carrousel Factory Museum provides a unique glimpse into the history of carousel manufacturing and is a fun destination for
families. Tonawanda Rails to Trails is a beautiful trail system ideal for biking and walking.
Ellicott Creek Park offers extensive recreational facilities, including picnic
areas, sports fields, and fishing spots. Lastly, the Historical Society of the
Tonawandas showcases the rich history of the area with fascinating exhibits and artifacts.
Each of these attractions offers unique experiences that highlight
Tonawanda’s cultural and natural heritage.
Choosing Insulation Depot USA for insulation services in Tonawanda is a wise decision for residents and businesses seeking reliable and efficient solutions.
The company offers a comprehensive range of services,
including insulation installation, soundproofing, and thermal insulation.
Insulation Depot USA’s commitment to quality workmanship and exceptional customer service ensures that all insulation needs are met promptly and professionally.
For those living in Tonawanda, Insulation Depot USA is the trusted partner for
maintaining an energy-efficient and comfortable living environment, providing peace of mind
and comfort throughout the year.
In Tonawanda, New York, maintaining proper insulation is crucial due
to the city’s changing seasons and the need for energy efficiency.
Insulation Depot USA offers essential insulation services such as attic insulation, wall insulation, and blown-in insulation, ensuring homes
and businesses in neighborhoods like Raintree Island and Cardinal O’Hara stay warm in the
winter and cool in the summer. The significance of having
a reliable insulation contractor in Tonawanda
cannot be overstated, as proper insulation is vital for
reducing energy costs and maintaining a comfortable indoor environment
throughout the year.
Insulation Depot USA operates in Tonawanda, a city founded in 1836.
Tonawanda is a historic city with a population of
14,991 as of 2022 and 6,947 households. The city is well-connected by Interstate 190,
a major highway that facilitates easy travel across the region. An interesting
fact about Tonawanda is its role in the early development of the Erie Canal, which significantly contributed to the city’s growth and prosperity.
Today, Tonawanda is known for its vibrant community and
diverse recreational opportunities, making it a great place to live and work.
Your articles are always so helpful.
Thank you for your sharing. I am worried that I lack creative ideas. It is your article that makes me full of hope. Thank you. But, I have a question, can you help me?
Your article helped me a lot, is there any more related content? Thanks!
Thanks for sharing. I read many of your blog posts, cool, your blog is very good.
Thank you for your sharing. I am worried that I lack creative ideas. It is your article that makes me full of hope. Thank you. But, I have a question, can you help me?
Excellent beat ! I would like to apprentice while you amend your site,
how can i subscribe for a blog site? The account helped me a acceptable deal.
I had been tiny bit acquainted of this your broadcast provided bright clear concept
Can you be more specific about the content of your article? After reading it, I still have some doubts. Hope you can help me.
The Best Premium IPTV Service WorldWide!
Good write-up, I am regular visitor of one’s website, maintain up the excellent operate, and It is going to be a regular visitor for a lengthy time.
UID_72942561###
Berita Hot! 🔥🔥 Isa Rachmatarwata Tersangka Kasus Jiwasraya Buntut kerugian negara hingga 16,8 Triliun! 😲😲
Your article helped me a lot, is there any more related content? Thanks!
UID_53550706###
Berita terbaru! 🔥 Isa Rachmatarwata Ditahan Kejagung dalam kasus Jiwasraya. 🚔👮♂️
UID_57092237###
Berita gembira! 🎉 Pasokan Gas 3 Kg di Kramat Jati Kembali Normal 🎊 Siap-siap belanja gas, ya! 💪🔥
UID_72339950###
Heboh! 📣🔥 Unjuk Rasa di Polda Jatim 📢 Menuduh Jokowi Terlibat Korupsi! 😱🔍
UID_27764099###
Ini yang di ganti >>> “Bingung Kenapa 😕❓” Federasi Sepak Bola Pakistan Disanksi FIFA “Baca Penyebabnya Disini! 🧐🔍”
UID_74743238###
Ini dia! 🎉🎉 ASN BKN Ubah Pola Kerja yang baru dan efisien! 🎉🎉.
UID_83344528###
Inilah 🎉🎉 Pupuk Inovatif Kebun Riset Kujang yang bikin Wamen BUMN bangga! 💪🏻🇮🇩
Hi, Neat post. There’s an issue along with your site in internet explorer, may check this… IE nonetheless is the marketplace chief and a good component of other people will miss your wonderful writing due to this problem.
Thank you for the good writeup. It in fact was a amusement account it. Look advanced to more added agreeable from you! By the way, how can we communicate?
UID_25978847###
situs terbaik hanya di slot gacor agentotoplay
UID_95859078###
Baru-baru ini, para pemain Mahjong Wins 3 dikejutkan dengan bocoran RTP yang diklaim bisa meningkatkan peluang kemenangan secara signifikan. Banyak yang percaya bahwa informasi ini membantu mereka mendapatkan hasil yang lebih konsisten dalam permainan. Jika Anda ingin tahu lebih lanjut, cek bocoran RTP Mahjong Wins 3 hari ini dan lihat apakah strategi ini benar-benar efektif.
UID_57331425###
Skandal terbaru mengguncang komunitas pecinta game slot setelah seorang admin terkenal, Rachel, dikabarkan tertangkap membocorkan pola kemenangan Gate of Olympus. Banyak yang bertanya-tanya apakah informasi ini benar-benar akurat atau hanya sekadar rumor yang beredar di kalangan pemain. Simak lebih lanjut detailnya dalam artikel admin Rachel tertangkap bocorkan pola Gate of Olympus.
UID_17136916###
Kisah inspiratif datang dari seorang tukang ojek online asal Tangerang yang berhasil membawa pulang hadiah fantastis setelah bermain Mahjong Ways 2. Dengan modal kecil, ia sukses mengubah nasibnya dan meraih kemenangan besar senilai 200 juta rupiah. Penasaran bagaimana caranya? Simak kisah lengkapnya di artikel tukang ojek online menang 200 juta dari Mahjong Ways 2.
UID_87427935###
Cek yuk! 🚍👮♂️Operasi Keselamatan 2025 Polres Ciamis Siapa tahu bus favoritmu jadi sasaran! 😱👍
UID_18563832###
Ini yang di ganti >>> Yuk, ketahui lebih lanjut tentang Syarat Gabung OECD dan Pentingnya Ratifikasi Konvensi Antisuap di sini! 🕵️♀️🔎📚.
UID_83003169###
Kenalan yuk! 🤝 Dengan guru inspiratif ini, Guru Dede Sulaeman yang mengajarkan cara merapikan pakaian di kelasnya. 👕👚🎓
UID_97211224###
Yuk, sehat bersama! 👨⚕️👩⚕️ Cek kondisi tubuh kamu di Program Cek Kesehatan Gratis sekarang juga! 💉🌡️ Selalu jaga kesehatan, ya! 🏥💖
You actually make it seem so easy with your presentation but I find this matter to be actually something which I think I would never understand. It seems too complex and extremely broad for me. I’m looking forward for your next post, I will try to get the hang of it!
Excellent read, I just passed this onto a friend who was doing some research on that. And he actually bought me lunch since I found it for him smile Therefore let me rephrase that: Thanks for lunch! “We steal if we touch tomorrow. It is God’s.” by Henry Ward Beecher.